We have compiled most frequently asked .NET Interview Questions which will help you with different expertise levels.
.NET Interview Questions on JQUERY
Question 1.
What is jQuery?
Answer:
jQuery is a reusable JavaScript library which simplifies JavaScriptcoding. So rather than writing lengthy JavaScript code as shown below you can use jQuery.
document.getElementByld(“txt1”).value = “hello”;
By jQuery the above JavaScript code is now simplified as shown below.
$(“#txt 1 ”). val(“Hello”);
Question 2.
So will jQuery replace JavaScript?
Answer:
No, jQuery is not meant to replace JavaScript. jQuery is a library while JavaScript is a language. jQuery sits on the top of JavaScript to make your development easy.
Question 3.
So how do we use these reusable jQuery libraries?
Answer:
You need to download jQuery.js file from jQuery.com and include the same in your Web pages. The jQuery files are named with version number like “jQuery-1.4.1.js” where 1.4.1 is the version of the JavaScript file. So at the top of your Web page you need to include the JavaScript as shown in the below code.
<script src-”Scripts/jQuery-1.4.1.min.js” type-”text/javascript”x/script>
Question 4.
What is CDN (Content Delivery Network)?
Answer:
In CDN multiple copies of the Website is copied on different geographical servers. When users send request for Website content which have CDN-enabled depending on their geographical location, content is served from the nearest geographical location server to the user.
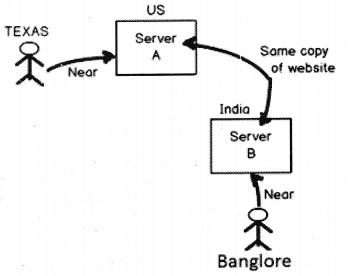
So if a user is from India, the Indian CDN server will serve request for Indian users. This leads to faster delivery of data.
Question 5.
For jQuery files which are the popular CDN’s?
Answer:
There are two popular CDN’s: Microsoft and Google.
If you want to reference Google CDN JQuery files you can use the below script.
<script type="text/javascript" .
src=''HTTP : //ajax, googleapis . com/aj ax/libs/jQuery/1.9.1/ j Query.min.j s " >
</script>
If you want to use Microsoft CDN you can use the below JavaScript.
<script type="text/javascript"
src="HTTP: //ajax.microsoft.com/ajax/jQuery/jQuery-1.9.1.min.j s">
</script>
Question 6.
How can we reference local jQuery files if CDN fails?
Answer:
Many times it is possible that Microsoft and Google servers can go down for some time. So in those situations you would like your page to reference jQuery files from local server.
So to implement a CDN fallback is a two-step process:
First reference is the CDN jQuery. In the below code you can see we have reference Microsoft CDN jQuery file.
HTTP: //ajax, microsoft. com/ajax/jQuery/jQuery-1.9.1. min.js “></script>
Now if Microsoft CDN is down then the jQuery value will be “undefined”. So you can see in the below code we are checking if the jQuery is having “undefined” value then do a document write and reference your local jQuery files.
if (typeof jQuery == 'undefined')
{
document.write(unescape("%3Cscript src='Scripts/jQuery.1.9.1.min.js' type='text/javascript'%3E%3C/script%3E"));
}
Below is the full code for the same.
<script type="text/javascript" src=" HTTP: //ajax.microsoft.com/ajax/jQuery/ jQuery-1.9.1.min.js "></script>
<script type=''text/javascript">
if (typeof jQuery == 'undefined')
{
document.write(unescape(”%3Cscript src='Scripts/jQuery.1.9.1.min.js'
type='text/javascript'%3E%3C/script%3E")); v
}
</script>
Question 7.
What is the difference between jQuery.js and jQuery.min.js file?
Answer:
First thing both the files provide the same jQuery functionalities. One is a long version and the other is compressed/minified version. The minified version is compressed to save bandwidth and space by compressing, and removing all the white spaces. Figure 7.2 shows the view of jQuery.js.
(function( window, undefined ) {
// Define a local copy of jQuery
var jQuery = function( selector, context ) {
// The jQuery object is actually just the init a return
new jQuery.fn.init( selector, context );
};
// Map over jQuery in case of overwrite
JQuery = window. jQuery,
// Map over the $ in case of overwrite
_$ = window.$,
Figure 7,3 shows the view of jQuery.min.js file (compressed and minified).
* Date: Mon 3 an 25 19:43:33 2010 -0500
*/
(function(z,vj{function la(){if(!c,isReady){try{r.dotuwentElement.doScroll e(a[0],b)multifunction
3(){return(new Date).getTime()}function Y(){returr a.currentTarget);m=0;for(s»i.length;sjm++)for(o in x)
{j=x[o];n=i[m].elefl lljfunction qa(a,b){var d=0;b.each(function(){if(this.nodeHa«e===(a[d]&&aj c.clean
(a,b,f,d)}if(e)c.fragments[a[0]]=i?f:l;return{fragment:f,cacheable: va»false,P=[],L,$=Obj ect.prototype.toSt
ring,a a=Obj ect.prototype.ha sOwnProf [f ]) ja=(a.cacheable?a.fragment.cloneNode(true):a.fragment).
childNodes}}el: this)},selector:"'Jquery:"1,4.1”,length:0,size:function(){return this.ler a,b)},ready:function(a)
{c.bindReady();if(c,isReady)a.call(r,c);else P&SP.f c.fn.init,prototype=c.fnj c.extend=c.fn.extend-function()
{var a=arguments[( Oa;if(a)z.JQuery=Naireturn c},isReady:false,ready:function(){if(ic.isReadj c.ready).;var
a=false|try{a=z.fraMeEle«ent==mill}c3tch(b){}r.docu*enfElemer
Figure 7.3: Compressed and Minified
Question 8.
When should we use jQuery.js over jQuery.min.js?
Answer:
When you are doing development use “jQuery.js” file because you would like to debug the JavaScript code, etc. Use “jQuery.min.js” for production environment. In production / live environment we would like to consume less bandwidth, we would like to our pages to load faster. .
Question 9.
What is the use jQuery.vsdoc.js?
Answer:
This file you can include if you want to enable Intellisense in Visual Studio for jQuery as shown in Figure 7.4.
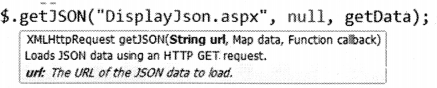
Question 10.
How does the basic syntax of JQuery looks like?
Answer:
jQuery syntax structure can be broken down into four parts:
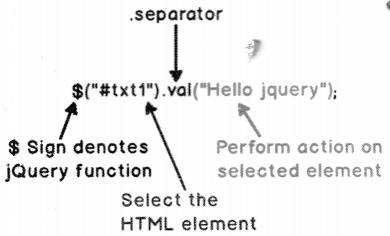
All JQuery commands start with a “$” sign as shown in Figure 7.5.
- Followed by the selection of the FITML element. For example below is a simple image where we are selecting a HTML textbox by id “txt1 ”.
Then followed by the dot (.) separator. This operator will separate the element and the action on the element.
- Finally what action you want to perform Figure 7.5: Basic Syntax
on the HTML element. For instance in the below jQuery code we are setting the text value to “Hello jQuery’.
Question 11.
What is the “$” sign in jQuery?
Answer:
The “$” sign is an alias for jQuery.
Question 12.
When should we use jQuery.noConflictQ?
Answer:
There are many JavaScript frameworks like MooTools, Backbone, Sammy, Cappuccino, Knockout, etc. Some of these frameworks also use “$” sign so this can lead to conflict with jQuery framework.
So you can use the “noConflict” method and release the jQuery “$” sign as shown in the below code.
$.noConflict();
jQuery(“p”).text(“i am jQuery and I am working... ”);
You can also create your own jQuery shortcut as shown below.
var jq = $.noConflict();
jq(“p”). text(“l am invoked using jQuery shortcut... ”);
Question 13.
What are the different ways by which you can select a HTML element in jQuery?
Answer:
You can select jQuery elements in the following ways:
Select all: Below is a simple code snippet which selects all elements and hides them.
$(“*”). hide( );
Select by ID: If your HTML elements have id’s like the below HTML code andyou want to select the same by id.
<p id="p 1 ">Some Para</p>
Below is how the jQuery code by ID selection code would look like.
$(“#p1”).hide();
Select by class: If your HTML element has class names and you want to select them in jQuery then you need to use the dot operator followed by the classname as shown in the below code.
<p class=”class1 ">Some Para</p> $(“.class1”).hide()
Select by class and element: Consider the below HTML code where we have elements with “class’
names.
<p class="cl">para 1</P>
<P class="cl">para 2</p>
<P class="c2">para 2</p>
<P class="c2">para 2</p>
So if you wish to select by “element” and “class” in jQuery your selector code becomes something as below.
$(“p.c1”).hide();
Select using Equal method
Select using Find method
Select using Filter method
Question 14.
What is the use of document.ready in jQuery?
Answer:
“document. ready” event occurs once the complete HTML DOM is loaded. So the next question is when do we actually need this event?. Consider the below simple code where we are trying to set a text box “textl” with value “Sometext”.
Now at the point when JQuery code tries set the textbox value, at that moment that text bcx is not available in the HTML DOM (Document Object Model). So it throws an exception for the same.
<script>
$("#textl").val("Sometext"); // Throws exception as the textbox is not //accessible at‘ this moment
</script>
</head>
<body>
<input type="text" id="textl11 />
</body>
So we would like to execute the JQuery code which sets the textbox value only when all the HTML objects are loaded in DOM. So you can replace the code of setting text box value to something as shown below.
<script>
$(document).ready(function(){
$("#textl").val("Sometext");
}) ;
</script>
Question 15.
Can we have two document. ready in a Web page?
Answer:
Yes.
Question 16.
How can we attach a method to a HTML element event using jQuery?
Answer:
Below is a simple code which attaches a function to click event of a button.
$("button").click(function(){
$("p").toggle();
}) ;
Below is one more example where we have attached the function to a mouseenter event of a paragraph.
$("#pl").mouseenter(function(){
alert("You entered pi!");
}) ;
Question 17.
How can we add a style using jQuery?
Answer:
$(“li”).filter(“.middle”).addClass(“seiected”);
<style>
.selected { color: red; }
</style>
Question 18.
What is JSON?
Answer:
JSON (JavaScript Object Notation) is a simple data exchange format which helps to communicate between JavaScript and server-side technologies like Servlets, JSP (Java Servlet Pages), WCF (Windows Communication Foundation), ASP.NET or Active Server Pages .NET etc.
The format of JSON is as shown below. The below format represents a “Customer” object with “CustomerCode” and “CustomerName” properties as shown in Figure 7.6.
{“CustomerCode”: “1001”, “CustomerName”: “Shiv”}
Now this format can be easily consumed by JavaScript and transformed into JavaScript object. Look at the Figure 7.6 where we have provided JSON to JavaScript variable and you can see how a JavaScript object is evaluated with “CustomerCode” and “CustomerName” properties.
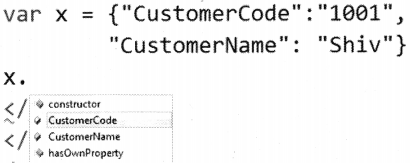
If you look at the Web architecture it has two parts: browser and server. On the browser side JavaScript is the most
prominent and well established language while on server-side you have different technologies like JSP, ASP.NET, PHP (a recursive backronym; HyperText Preprocessor) etc.
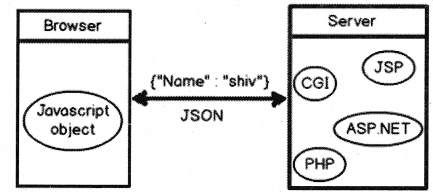
So if the server-side technologies emit out JSON format which can be easily transformed into a JavaScript object that would really ease the communication between multiple server-side technologies and JavaScript language. Figure 7.7: Web Architecture
Question 19.
How to convert string to JSON object?
Answer:
“JSON.parse” helps us to convert string to JSON object.
var str = ‘{ “Name”: “Shiv”, “Salary”: “1000”}’;
var Customer = JSON.parse(str); alert(Customer.Name);
Question 20.
Was not SOAP meant to do the same thing which JSON does?
Answer:
SOAP is heavy due to XML tags. For example, a SOAP message “<Name>shiv</Name>” will become short, sweet and light in JSON like “Name”: “Shiv”. Second most important it evaluates as JavaScript object. To convert the complicated SOAP XML (extensible Markup Language) as shown in Figure 7.8 in to javascript JSON object would be a tough and tedious task.
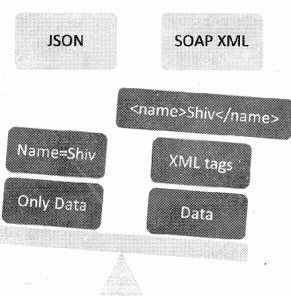
Question 21.
Do all technologies support JSON?
Answer:
Yes, almost all technologies which deal with exchange of data support JSON. For instance if you want to that your WCF service should send JSON message rather than SOAP
you can set the “ResponseFormat ” as “WebMessageFormat. Json” on your operation contract.
[OperationContract]
[Weblnvoke(Method="GET", • UriTemplate="/
GetData",
RequestFormat=WebMessageFormat.Json,
ResponseFormat=WebMessageFormat.Json)]
string GetData();
If you want your MVC to emit out JSON data you can return ” JsonResult” as shown below. If you call the below action it will emit out Customer objects in JSON format.
public JsonResult CustomerJson()
{
List<Customer> obj1 = new List<Customer>( );
Thread.Sleep(5000);
Customer obj = new customer ( );
obj.CustomerCode = "1001";
obj1.Add(obj);
return Json(objl, JsonRequestBehavior.AllowGet);
}
If you want to emit JSON using ASP.NET we need to use the “DataContractJsonSerializer” class as shown in the below code. “myPerson” is the class.
DataContractJsonSerializer serializer = new
DataContractJsonSerializer(myPerson.GetType());
MemoryStream ms = new MemoryStream();
serializer.WriteObject(ms, myPerson);
string json = System.Text.Encoding.UTF8.GetString(ms.ToArray( ));
Response.Clear();
Response.ContentType = "application/json;charset=utf-8";
Response.Write(json);
Response.End( );
Question 22.
How can you make a JSON call using jQuery?
Answer:
Let’s assume you have a MVC controller action “getEmployee” which emits out employee JSON object as shown in the below code. Please note you can always emit JSON from any server technology like WCF, ASP.NET, MVC, etc., as discussed in the previous questions.
public JsonResult getEmployee()
{
Emp obj = new Emp();
obj.empcode = "1001";
return Json(obj, JsonRequestBehavior.AllowGet) ;
}
To make a call to the above MVC action using jQuery we need to use "get JSON" method. Below is the simple code for the same. It has three parameters:
1. The first parameter is the URL which emits out JSON. For instance in the below code the URL is
"/Employee/getEmployee".
2. The next parameter helps us to pass data to the resource which emits out JSON currently it’s the MVC action. The second parameter is NULL for now.
3. The last parameter is the callback function which will be invoked once the MVC action returns data. You can see how the “getData" function just displays the "empcode" property. Because the output is in JSON it automatically converts the JSON data to JavaScript object.
$.getJSON("/Employee/getEmployee", null, getData); function getData(data)
{
alert(data.empcode);
}
Question 23.
How can we post JSON to Server?
Answer:
We can use the “post” method of jQuery to send data to the server. Below is how the post method call looks like. First parameter is the URL which will accept JSON data, second is the data which we want to send and the final parameter is the callback function where we receive the response.
var mydata ={name: "Shiv", city: "Mumbai"};
$.post("/Send/Request", // URL
mydata, // Data to be sent
function(data, status){alert(data + " " + status);}); // Call back function
Question 24.
How can we post a complete HTML form in JSON format?
Answer:
To post a complete HTML form we need to call “serialize” function as shown in the below code. “form1” is a HTML form. The data given by the function can then be passed to the “post” method of jQuery. “DisplayData” is a callback function to handle the output given by the server.
var Mydata = $(“#form 1 ”). serialize( );
$.post(“/Customer/getCustomer”, JSON. stringify(MyData), DisplayData);
The above posted JSON string is received at the server side “request.inputstream”, below is a simple sample code for the same.
System.10.Stream body = Request.inputstream;
System.10.StreamReader reader = new System.10.StreamReader(body);
string s = reader.ReadToEnd() ;
Question 25.
How can we convert JSON string into C# object?
Answer:
To convert a JSON string to a C# object we need to use the ” JavascriptSerializer” class as shown in the below code.
“JsonString” is the string which has the JSON value and by using “Deserialize” we are converting the string to a C# object. Now this object which we receive is a collection of “key” and “value” pair which can be browsed and accessed in C#.
var jsonser = new JavaScriptSerializer()
var obj = jsonser.Deserialize<dynamic>(JsonString);
foreach (var x in obj)
{
String strvalue = x["value"];
}
Question 26.
What are Single Page Applications (SPA)?
Answer:
SPA means your Web page has the following features:
- Utilize the browser client power to the maximum by executing the maximum code on the client- side by using JavaScript, HTML and CSS.
- Rather than loading the complete page necessary HTML fragments or JSON data is loaded as the user demands.
- JavaScript which handles DOM manipulation, binding, Ajax calls are separated into controllers thus separating views and models.
- DOM manipulations are replaced by declarative programming.