Web forms interview questions: We have compiled most frequently asked .NET Interview Questions which will help you with different expertise levels.
.NET Interview Questions on ASP.NET WebForms
Question 1.
What are the different events which fire the ASP.NET page life cycle?
Answer:
Following is the sequence in which the events occur:
- Init
- Load
- Validate
- Event
- Render
Note: Remember the word SILVER: SI (Init) L (Load) V ( Validate)E ( Event) R ( Render).
Pagejnit event only occurs when the first time the page is started, but Page Load occurs in the subsequent requests of the page.
Question 2.
What are HTTP Handlers and HTTP Modules?
Answer:
Handlers and modules help you inject pre-processing logic before the ASP.NET request reaches the Website. One of the scenarios where you would like to use them is before the request reaches the server you would like to check if the user has authenticated or not.
Question 3.
What is the difference between HTTP Handlers and HTTP Modules?
Answer:
HTTP Handler is an extension-based processor. In other words, the pre-processing logic is invoked depending on file extensions.
HTTP Module is an event-based processor. In other words, ASP.NET emits a lot of events like begin request, AuthenticateRequest, etc., we can write login functionality in those events using HTTP Module.
Question 4.
How do we write an HTTP Handler?
Answer:
• Create a class and implement “iHTTPHandler”.
public class clsHTTP Handler: IHTTP Handler { public bool IsReusable { get { return true; } } public void ProcessRequest(HTTPContext context) { // Put implementation here. } }
- Define implementation for “ProcessRequest” method.
- Register the class in Web.config with file extension in HTTPHandlers tag.
<HTTP Handlers>
<add verb=”*” path=”*.gif” type=”clsHTTP Handler”/>
</HTTP Handlers>
Question 5.
How do we write an HTTP Module?
Answer:
- Create a class and implement iHTTPModuie interface.
- Attach events with your methods and put the implementation in those events as shown in the below code snippet.
public class clsHTTP Module: IHTTP Module { public void Init(HTTPApplication context) { this.HTTPApp = context; HTTPApp.Context.Response.Clear( ); HTTPApp.AuthenticateRequest += new EventHandler (OnAuthentication); HTTPApp.AuthorizeRequest += new EventHandler(OnAuthorization); HTTPApp.BeginRequest += new EventHandler(OnBeginrequest); HTTPApp.EndRequest += new EventHandler(OnEndRequest); HTTPApp.ResolveRequestCache += new EventH } void OnUpdateRequestCache(object sender, EventArgs a) { //Implementation } void OnReleaseRequestState(object sender, EventArgs a) { //Implementation } void OnPostRequestHandlerExecute(object sender, EventArgs a) { //Implementation } void OnPreRequestHandlerExecute(object sender, EventArgs a) { //Implementation } void OnAcquireRequestState(object sender, EventArgs a) { //Implementation } void OnResolveRequestCache(object sender, EventArgs a) { //Implementation } void OnAuthorization(object sender, EventArgs a) { //Implementation } void OnAuthentication(object sender, EventArgs a) { //Implementation } void OnBeginrequest(object sender, EventArgs a) { //Implementation } void OnEndRequest(object sender, EventArgs a) { //Implementation } }
- Register the class in Web.config in HTTPModules tag as shown in the below code snippet.
<HTTP Modules>
<add name=”clsHTTP Module” type=”clsHTTP Module”/>
</HTTP Modules>
Question 7.
Can you explain how the ASP.NET application life cycle works?
Answer:
Note: When anyone asks for page life the SILVER answer is appropriate, but when the interviewer is asking for the application life cycle, two more things come into the picture handlers and modules.
Below is how ASRNET events fire.
- First the HTTPModule events like BeginRequest, AuthenticateRequest, fire.
- Then the HTTPHandlers fires if the file extension matches the request page name.
- Finally Page events fire, i.e., init, load, validate, event, and render.
Question 8.
in which event are the controls fully loaded?
Answer:
Page load event guarantees that all controls are fully loaded. Controls are also accessed in Page_init events but you will see that the view state is not fully loaded during this event.
Question 9.
What is postback in ASP.NET and what is the IsPostBack property?
Answer:
Postback occurs when data is posted to the page. Now, this data posting can happen when someone clicks on the Submit button or some events occur on Ulcontrols.
If you want to detect that the page is called because someone posted data then we need to check the “isPostback” property of the page object. If someone has called the page by just putting a URL (Uniform Resource Locator) or hitting refresh then this property is set to false, but if someone has posted data by clicking on a Submit button then this property is set to true.
Question 10.
Can you explain “AutoPostBack”?
Answer:
Postback happens when you click submit buttons on an ASP.NET page.
But post back does not happen:
- When you click checkboxes.
- When data changes in combo boxes.
- When someone types on the textbox and presses Enter key or Tab.
So in case you want that postback happens when regular control data changes then you need to set the AutPostBack property to true on the controls. For instance, in the below image we are setting the textbox AutoPostBack property to true.
So now someone types any content on the textbox and presses tab/enter postback happens.
Question 11.
What is the use of @ Register directives?
Answer:
@ Register directive informs the compiler of any custom server control added to the page.
Question 12.
What is the use of Smart Navigation property?
Answer:
It is a feature provided by ASRNET to prevent flickering and redrawing when the page is posted back.
Question 13.
What is AppSetting Section in the “Web.Config” file?
Answer:
Web.config file defines configuration for a Web project. Using “AppSetting” section, we can define user-defined values. Example below is a “Connection String” section, which will be used throughout the project for database connection.
<Configuration>
<appSettings>
<add key=”ConnectionString” value=”server=xyz;pwd=www;database=testing”/>
</appSettings>
Question 14.
Where is View State information stored?
Answer:
In HTML hidden fields.
Question 15.
How can we create custom controls in ASP.NET?
Answer:
User controls are created using.ASCX in ASP.NET. After.ASCX file is created you need to do two things in order that the ASCX can be used in the project:
- Register the ASCX control on the page using the percentage@ Register directive. Example
<% @ Register tag prefix=”Accounting” Tag name=”footer” Src=”Footer.ascx” %>
- Now to use the above accounting footer on the page you can use the below directive.
<Accounting: footer runat="server” />
Question 16.
How many types of validation controls are provided by ASP.NET?
Answer:
There are six main types of validation controls:
RequiredFieldValidator: It checks whether the control has any value. It is used when you want the control should not to be empty.
RangeValidator: It checks if the value in validated control is in that specific range. Example: TxtCustomerCode should not be more than eight in length.
CompareValidator: It checks that the value in controls should match some specific value. Example: Textbox TxtPie should be equal to 3.14.
RegularExpressionValidator: When we use the control, the value should match with a specific regular expression.
CustomValidator: It is used to define user-defined validation.
Validation Summary: It displays a summary of all current validation errors on an ASP.NET page.
Question 17.
How can we force all the validation control to run?
Answer:
Page.Validate
Question 18.
How can we check if all the validation control are valid and proper?
Answer:
Using the Page. isValid ( ) property you can check whether all the validations are done.
Question 19.
If client-side validation is enabled, will server-side code still run?
Answer:
When client-side validation is enabled server emits JavaScript code for the custom validators. However, note that does not mean that server-side checks on custom validators do not execute. It does this redundant check two times, as some of the validators do not support client-side scripting.
Question 20.
Which JavaScript file is referenced for validating the validators at the client side?
Answer:
WebUIValidation.js JavaScript file installed at “aspnet_client” root IIS (Internet Information Services) directory is used to validate the validation controls at the client-side
Question 21.
How to disable client-side script in validators?
Answer:
Set ‘ EnableClientScript’ to false.
Question 22.
How can I show the entire validation error message in a message box on the client-side?
Answer:
Invalidation summary set ” show message box” to true.
Question 23.
If validation is very complex what will you do?
Answer:
Best is to go for CustomValidator. Below is a sample code for a custom validator, which checks that a textbox should not have zero value
<asp: CustomValidator id="CustomValidatorl" runat="server" ErrorMessage="Number not divisible by Zero" ControlToValidate="txtNumber" OnServerValidate="ServerValidate" ClientValidationFunctron="CheckZero" /><br> Input: <asp: TextBox id="txtNumber" runat="server" /> <script language="javascript"> <!—function CheckZero(source, args) { int val = parselnt(args.Value, 10); if (value==0) { args . IsValid = false; } } // -> </script>
Question 24.
How can you enable automatic paging in the data grid?
Answer:
Following are the points to be done in order to enable paging in the data grid:
- Set the “Allow Paging” to true.
- In the PagelndexChanged event, set the current page index clicked.
Question 25.
What is the use of the “GLOBAL.ASAX” file?
Answer:
It allows to executeASP.NET application-level events and sets application-level variables.
Question 26.
What is the difference between “Web. config” and “Machine. config”?
Answer:
“Web. config” files apply settings to each Web application, while the “Machine. config” file applies settings for all ASP.NET applications running on that machine.
Question 27.
Can we have two “Web. config” files in one Web application?
Answer:
Yes, you can have two Web.config files in a Web application.
Question 28.
In what scenarios will a project have two Web.config files?
Answer:
When developers develop applications they divide projects into modules and many times these modules are hierarchically connected. For instance, we are creating a Web application for an organization that has departments.
We would like to divide our project source code into modules as shown below. And every module will have its own configuration. Figure 5.2 shows a simple Web application project with two modules “Accounts” and “Sales”.
So the main organization site has a config file at the root level followed by config files inside each module.
So let’s say if you are browsing “Home.aspx” from Sales folder then “Web.config” file in the sales folder will get precedence. If the configuration is not found then it falls back to the “Web.config” file of the main directory.
Question 29.
Can ASP.NET project run without a Web. config file?
Answer:
Yes.
Question 30.
What are SESSION and APPLICATION objects?
Answer:
Session objects store information between HTTP (Hypertext Transfer Protocol) requests for a particular user, while application objects are global across users.
Question 31.
What is the difference between ‘Server. Transfer’ and ‘Response. Redirect’?
Answer:
Following are the major differences between them:
‘Response.Redirect’ sends message to the browser saying it to move to some different page, while ‘Server.Transfer’ does not send any message to the browser but rather redirects the user directly from the server itself. So in ‘Server.Transfer’ there is no round trip while ‘Response.Redirect’ has a round trip and hence puts a load on server.
Using ‘Server.Transfer’ you cannot redirect to a different from the server itself. For example, if your server is www.yahoo.com you cannot use ‘Server.Transfer’ to move to www.microsoft.com but yes, you can move to www.yahoo.com/travels, i.e., within Websites. Cross server redirect is possible only by using Response. Redirect.
With ‘Server.Transfer’ you can preserve your information. It has a parameter called as “preserveForm”. Therefore, the existing query string, etc. will be able in the calling page. If you are navigating within the same Website use “Server. Transfer” or else go for “Response. Redirect ( )”
Question 32.
What is the difference between authentication and authorization?
Answer:
This can be a tricky question. These two concepts seem altogether similar but there is a wide range of difference. Authentication is verifying the identity of a user and authorization is process where we check does this identity have access rights to the system. Authorization is the process of allowing an authenticated user access to resources.
Question 33.
What is impersonation in ASP.NET?
Answer:
By default, ASRNET executes in the security context of a restricted user account on the local machine. Sometimes you need to access network resources, such as a file on a shared drive, which requires additional permissions. One way to overcome this restriction is to use impersonation. With impersonation, ASP.NET can execute the request using the identity of the client who is making the request, or ASRNET can impersonate a specific account you can specify the account in Web.config.
Question 34.
What are the various ways of authentication techniques in ASP.NET?
ANswer:
There are three major ways of doing authentication and authorization:
• Windows: In this mode the users are stored in windows local user groups.
• Forms: In this mode we create a login screen and use the forms authentication class for validations. It’s a ticket-based authentication.
• Passport: In this mode the users are validated from Microsoft sites like Hotmail, devhood, MSN (Microsoft Network), etc., ticket is generated and that ticket can be used authentication and authorization process in your Web application.
Question 35.
Can you explain Forms authentication in detail?
Answer:
In old ASP if you are said to create a login page and do authentication you have to do a lot of custom coding. Now in ASRNET that has been made easy by introducing forms authentication. So let us see in detail what form authentication is.
Forms authentication uses a ticket cookie to see that user is authenticated or not. That means when user is authenticated first time a cookie is set to tell that this user is authenticated. If the cookies expire then Forms authentication mechanism sends the user to the login page.
Following are the steps, which defines steps for Forms authentication:
- Configure Web.config file with forms authentication tag. As shown below in the config file you can see we have give the cookie name and loginuri page.
<configuration> <system.Web> <!— Other settings omitted. —> <authentication mode="Forms"> <forms name="logincookies" loginTJrl = " login. aspx" protection="All" timeout="30" path="/" /> </authentication> </system.Web> </configuration>
- Remove anonymous access to the IIS Web application, following are changes done to Web.config file.
<configuration> <system.Web> <!— Other settings omitted. —> <authorization> <deny users="?" /> </authorization> </system.Web> </configuration>
- Create the login page, which will accept user information. You will have to create your login page that is the log in.aspx, which will actually take the user data.
- Finally a small coding in the login button.
Let us assume that the login page has two textboxes ‘ txtName’ and ‘ txtPassword’.
Also, import System.Web.Security and put the following code in login button of the page.
If Page.IsValid Then
If FormsAuthentication.Authenticate(txtName.Text, txtPassword.Text) Then
FormsAuthentication.RedirectFromLoginPage(txtName.Text, False)
Else
lblStatus.Text = “Error not proper user”
End If
End If
Question 36.
How do I sign out in forms authentication?
Answer:
FormsAuthentication.Signout( )
Question 37.
If cookies are disabled how will forms authentication work?
Answer:
It will pass data through query strings.
Question 38.
How do we implement windows authentication?
Answer:
- Create users in your local windows user group.
- In Web.config file set the authentication mode to windows.
- In Web.config file set <deny users=”?”/>.
Once you do the above three steps yourAST.NET pages will be authenticated and be authorized from the users stored in the windows local user group.
Question 39.
How can we do a single sign-on in ASP.NET?
Answer:
For single sign-on, we need to use forms authentication and put the same machine key in the Web. config files of all Web applications who will participate in single sign-on.
Question 40.
Can you explain membership and role providers in ASP.NET 2.0?
Answer:
A membership and role provider helps to automate your authentication and authorization code. When we want to implement user authentication and authorization in any project following are the routine task:
- Creation of user and roles tables.
- Code-level implementation for maintaining those tables.
- The user interfaces for ‘userid’ and ‘password’.
All the above tasks are automated using membership and roles.
In order to implement membership and roles run aspnet_regsql.exe from ‘C: \WINDOWS\Microsoft.NETAFramework\v2.0.50727’ folder.
This will create necessary readymade tables like users, roles, etc. You can then use the readymade API (Application Programming Interface) of membership and roles to create user and roles as shown in the Figure 5.4, and Figure 5.5, respectively.
Question 41.
Can you explain master pages concept in ASP.NET?
Answer:
Master pages are templates which can be applied to your Web pages to bring in consistent look and feel and uniform structure. By creating templates we also avoid a lot of duplication of code across Web pages.
For example, let’s say you want all your Web pages in your application to have left menu, banner and footer as shown in Figure 5.6. So you can create a template and then apply that template to your Web pages.
Question 42.
So how do you create master pages?
Answer:
To create a master page you can use the “Master Page” template from Visual Studio as shown in the Figure 5.7. In order to apply the master template we can use “Web Form using Master Page” template.
In the master page you can define placeholder using “ContentPlaceHolder” control. This is the place where yourASP.NET pages will be plug-in their data.
<body> This is the common template <asp: ContentPlaceHolder ID="ContentPlaceHolderl" runat="server"> </asp: ContentPlaceHolder> </body>
Once the master page template is created with the necessary placeholders. You can then later in the Web pages use the “MasterPageFile” to refer to the template and you can use the content placeholder to plug data as shown in Figure 5.8.
For instance you can see the below code snippet of a page which uses master pages. You can also see how the “ContentPlaceHolderl” is used to place a simple text data “Company was established in 1990”.
<%@ Page Title="" Language="C#" MasterPageFile="-/WebSiteTemplate.Master" AutuEventWireup="true" CodeBehind="Aboutus.aspx.cs" Inherits="MasterPageDemo. Aboutus" %> <asp: Content ID="Contentl" ContentPlaceHolderID="ContentPlaceHolder1"runat="server"> <P> Company was established in 1990</p> </asp: Content>
Note: Also refer video from the DVD “What are Master pages?”
Question 43.
What is the concept of Web parts?
Answer:
Web part is a window of information inside Web pages that can be edited, closed, minimized, dragged, and customized by the end-user. Web parts are all about providing customization to the end-user. Some of the customization features you can give to the end-user by using Web parts are as follows:
- You can add or remove control from the Web page.
- End-user can modify the appearance of the control.
- Controls can be moved to different locations on the Web page.
Figure 5.9 shows how the end-user is provided the ability to interchange the calendar and the login control on the fly.
Figure 5.10 shows one more magic of Web parts. End-user can edit appearance information of the Web part which has a calendar control inside.
The above customization either can be set at a personal level or for all users.
Question 44.
What are partial classes in ASP.NET?
Answer:
A partial class allows a single class to be divided into two separate physical files. During compile time these files get compiled into a single class. For instance, you can see in Figure 5.11 we have the customer class divided into two different files “customer1 .cs” and “customer2.cs”.
The above customization either can be set at a personal level or for all users.
Figure 5.10 shows one more magic of web parts. End-user can edit appearance information of the web part which has a calendar control inside.
Question 45.
What are partial classes in ASP.NET?
Answer:
A partial class allows a single class to be divided into two separate physical files. During compile time these files get compiled into a single class. For instance, you can see in Figure 5.11 we have the customer class divided into two different files “customer1 .cs” and “customer2.cs”.
During compilation, these files get compiled into a single class internally. So when you create an object of the customer class you will be able to see methods lying in both the physical files. For instance, you can see the “Add( ) ” method belongs to “customer1.cs” and the “Delete ( )” method belongs to “customer2.cs”, but when the customer object is created we can see both “Add ()” and “Delete ()” methods.
Question 46.
What is the difference between data grid and grid view?
Answer:
Grid view is a successor of data grid with the following benefits:
- Grid view has automatic paging as compared to the data grid where you need to write some code for paging.
- Additional column types and rich design-time capabilities.
Question 47.
What is the difference between grid view, data list, and repeater?
Answer:
Grid view and data grid by default display all the data in tabular format, i.e., in table and rows. The developer has no control to change the table data display of the data grid.
Data list also displays data in a table but gives some flexibility in terms of displaying data row-wise and column-wise using the repeat direction property.
Repeater control is highly customizable. It does not display data in the table by default. So you can customize from scratch the way you want to display data.
Question 48.
From a performance point of view, how do they rate?
Answer:
The repeater is fastest followed by Datalist/Gridview and finally data grid.
Question 49.
What is the method to customize columns in the data grid?
Answer:
Use the template column.
Question 50.
How can we format data inside the data grid?
Answer:
Use the DataFormatString property.
Question 51.
How to decide to select a data grid, data list, or repeater?
Answer:
Many make a blind choice of choosing a data grid directly, but that is not the right way.
Data grid provides the ability to allow the end-user to sort, page, and edit its data. However, it comes at a cost of speed. Second, the display format is simple in the form of rows and columns.
With its templates, the data list provides more control over the look and feel of the displayed data than the data grid. It offers better performance than a data grid.
Repeater control allows for complete and total control. With the repeater, the only HTML (HyperText Markup Language) emitted is the values of the data binding statements in the templates along with the HTML markup specified in the templates—no “extra” HTML is emitted, as with the data grid and data list. By requiring the developer to specify the complete generated HTML markup, the repeater often requires the longest development time. However, repeater does not provide editing features like data grid so everything has to be coded by the programmer. However, the repeater provides the best performance of the three data Web controls. The repeater is fastest followed by the data list and finally the data grid.
Question 52.
What are major events in GLOBAL.ASAX file?
Answer:
The GLOBAL.ASAX (also known as ASP.NET application file) file, which is derived from the HTTPApplication class, maintains a pool of HTTP Application objects and assigns them to applications as needed. The GLOBAL.ASAX file contains the following events:
Application not: Fired when an application initializes or is first called. It is invoked for all HTTP Application object instances.
Application Error: Fired when an unhandled exception is encountered within the application.
Application Start: Fired when the first instance of the HTTP Application class is created. It allows you to create objects that are accessible by all HTTP Application instances.
Application End: Fired when the last instance of an HTTP Application class is destroyed. It is fired only once during an application’s lifetime.
Session Start: Fired when a new user visits the application Website.
Session End: Fired when a user’s session times out, ends or leaves the application Website.
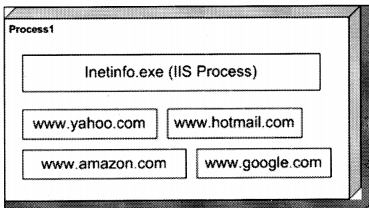
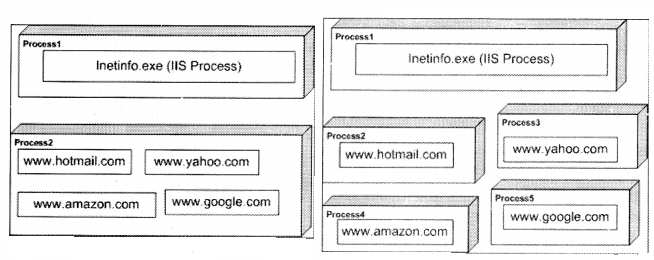
- In ASPX page you have to add itemTemplate tag in data grid.
<ItemTemplate> <asp: CheckBox id="CheckBoxl" runat="server" AutoPostBack="True" OnCheckedChanged="Check_Clicked"></asp: CheckBox> </ItemTemplate>
Protected Sub Check Clicked (By Val sender As Object, By Val e As EventArgs) 'Do something End Sub
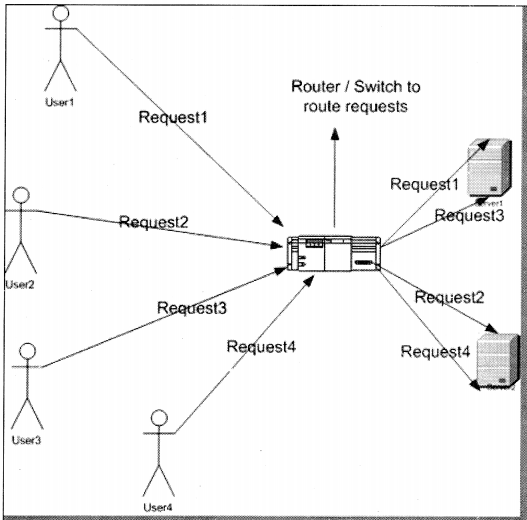
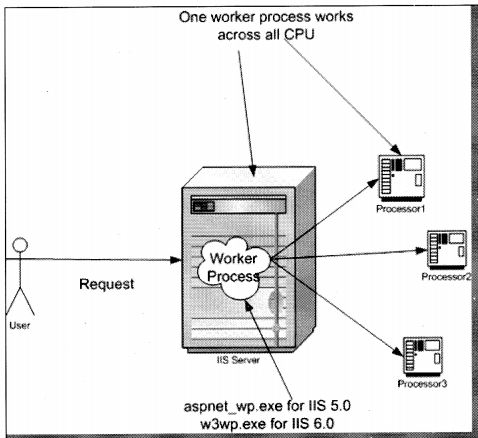
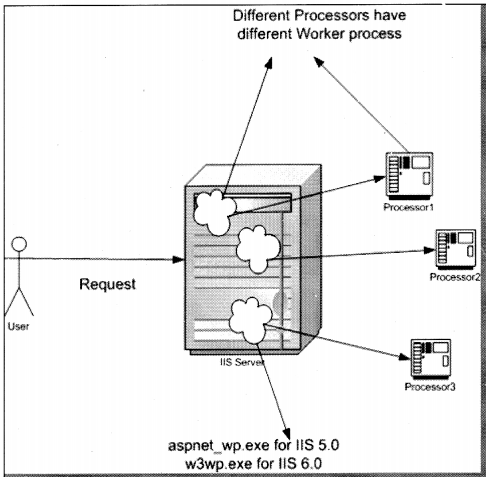
<processModel enable="true" timeout="infinite" idle Timeout="infinite" shutdown Timeout="0: 00: 05" requestLimit="infinite" requestQueueLimit="5000" memoryLimit="80” WebGarden="false" cpuMask="12 11 userName=" " password=" " logLevel="errors" clientConnectedCheck="0: 00: 05" />
- Click Start and then click Run.
- Type calc.exe and then click OK.
- Go to the View menu, click Scientific.
- Go to the View menu, click Binary.
- Use zero and one to specify the processors ASP.NET can or cannot use.
- On the View menu, click Decimal. Note the decimal number.
- Open the Web. config or machine.config file in a text editor, such as Notepad. The Web. config file is located in the folder where the application is saved.
- In the Web.config file, add the process Model configuration element under the System.Web element. Before adding <processModel> to Web.config file, the user has to make sure that the allowDefin.ition attribute in the <processModel> section of the Web.config file is set to everywhere.
- Add and then set the webGarden attribute of the processModel element to True.
- Add and then set the cpuMask attribute of the processModel element to the result that is determined in your calculation.
<processModel enable="true" WebGarden="true" cpuMask="12" />
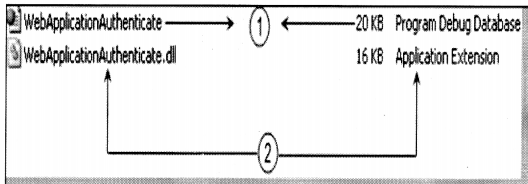
Note: There is also a fundamental difference in thinking when we need to use trace and when need to debug. Tracing is a process about getting information regarding program’s execution. On the other hand debugging is about finding errors in the code.
<trace enabled=”true" requestLimit=”10" pageOutput=”false” localOnly=”true”/>
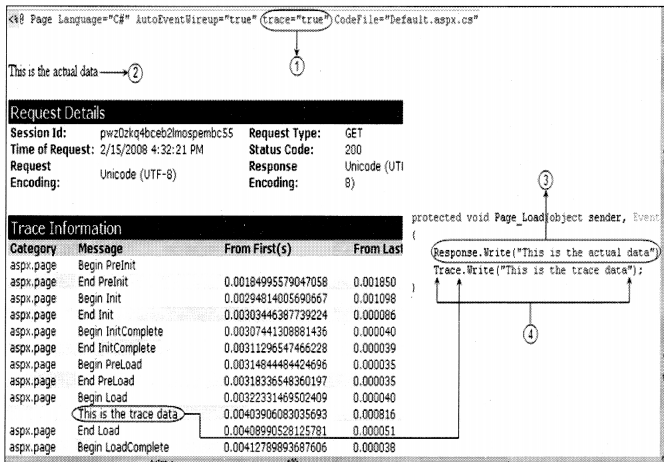
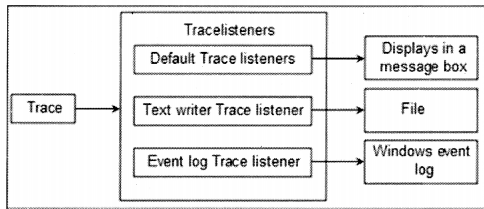
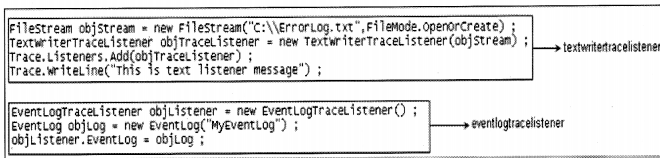
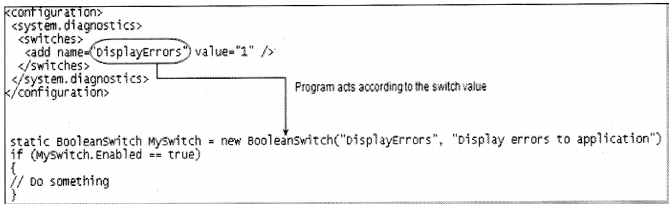
TraceSwitch objSwitch = new TraceSwitch("TraceWarningandError", "Error in trace") ; objSwitch.Level = TraceLevel.Warning ;
The Cache object is defined in the ‘System.web. Caching’ namespace. You can get a reference to the Cache object by using the Cache property Of the HTTPContext class in the ‘ System. Web’ namespace or by using the Cache property of the Page object.
Question 72.
What are dependencies in cache and types of dependencies?
Answer:
When you add an item to the cache, you can define dependency relationships that can force that item to be removed from the cache under specific activities of dependencies. For example, if the cache object is dependent on file and when the file data changes you want the Cache object to be update. Following are the supported dependency:
- File dependency: This allows you to invalidate a specific cache item when a disk based file or files change.
- Time-based expiration: This allows you to invalidate a specific cache item depending on predefined time.
- Key dependency: This allows you to invalidate a specific cache item depending when another cached item changes.
Question 73.
Can you write a simple code showing file dependency in cache?
Answer:
PartialClass Default_aspx PublicSub displayAnnouncement( ) Dim announcement AsString If Cache("announcement") IsNothingThen Dim file AsNew _ System.10.StreamReader _ (Server.MapPath("announcement.txt")) announcement = file.ReadToEnd file.Close( ) Dim depends AsNew _ System.Web.Caching.CacheDependency _ (Server.MapPath("announcement.txt")) Cache.Insert("announcement", announcement, depends) Endlf Response.Write(CType(Cache("announcement"), String)) EndSub PrivateSub Page_Init(ByVal sender AsObject, ByVal e As System.EventArgs) HandlesMe.Init displayAnnouncement() EndSub End Class
Above given method displayAnnouncement () displays banner text from announcement.txt file which is lying in Application path of the Web directory. Above method, first checks whether the Cache object is nothing, if the Cache object is nothing then it moves further to load the cache data from the file. Whenever the file data changes the Cache obj ect is removed and set to nothing.
Question 74.
What is Cache Callback in Cache?
Answer:
Cache object is dependent on its dependencies, for example file-based, time-based etc. Cache items remove the object when cache dependencies change. ASP.NET provides capability to execute a callback method when that item is removed from cache.
Question 75.
What is scavenging?
Answer:
When server running yourASP.NET application runs low on memory resources, items are removed from cache depending on cache item priority. Cache item priority is set when you add item to cache. By setting the cache item priority controls, the items scavenging are removed according to priority.
Question 76.
What are different types of caching using Cache object of ASP.NET?
Answer:
You can use two types of output caching to cache information that is to be transmitted to and displayed in a Web browser:
• Page Output Caching: Page output caching adds the response of page to Cache object. Later when page is requested page is displayed from cache rather than creating the page object and displaying it. Page output caching is good if the site is fairly static.
• Page Fragment Caching: If parts of the page are changing, you can wrap the static sections as user controls and cache the user controls using page fragment caching.
Question 77.
How can you cache different versions of same page using ASP.NET Cache object?
Answer:
Output cache functionality is achieved by using “OutputCache” attribute on ASP.NET page header. Below is the syntax.
<%@ OutputCache Duration-”20" Location=”Server” VaryByParam=”state” VaryByCustom=”minorversion” VaryByHeader=”Accept-Language”%>
- varyByParam: Caches different versions depending on input parameters sent through HTTP POST/GET.
- varyByHeader: Caches different versions depending on the contents of the page header.
- varyByCustom: Lets you customize the way the cache handles page variations by declaring the attribute and overriding the GetvaryByCustomstring handler.
- varyByControl: Caches different versions of a user control based on the value of properties of ASP objects in the control.
How will implement page fragment caching?
Page fragment caching involves the caching of a fragment of the page, rather than the entire page. When
portions of the page are need to be dynamically created for each user request, and this is best method as compared to page caching. You can wrap Web Forms user control and cache the control so that these portions of the page do not need to be recreated each time.
Question 78.
Can you compare ASP.NET sessions with classic ASP?
Answer:
ASP.NET session caches per user session state. It basically uses “HTTPSessionState” class. Following are the limitations in classic ASP sessions:
• ASP session state is dependent on IIS process very heavily. So if IIS restarts ASP session variables are also recycled. ASP.NET session can be independent of the hosting environment thusASP.NET session can be maintained even if IIS reboots.
• ASP session state has no inhered so’^tion to work with Web Farms. ASP.NET session can be stored in state server and SQL Server which can support multiple servers.
• ASP session only functions when the browser supports cookies.ASP.NET session can be used with browser side cookies or independent of it.
Question 79.
Which are the various modes of storing ASP.NET session?
Answer:
• InProc: In this mode, session, state is stored in the memory space of the Aspnet_wp.exe process. This is the default setting. If the IIS (Internet Information Services) reboots or Web application restarts then session state is lost.
• StateServer: In this mode session state is serialized and stored in a separate process (Aspnet_state.exe); therefore, the state can be stored on a separate computer (a state server).
• SQL Server: In this mode session state is serialized and stored in a SQL Server database. Session state can be specified in <sessionstate> element of application configuration file. Using State Server and SQL SERVER session state can be shared across Web Farms but note this comes at speed cost as ASP.NET needs to be serialized and deserialized data over network repeatedly.
Question 80.
Do sessions use cookies?
Answer:
Yes, sessions use cookies. The session id value is stored in the browser client temp directory. This id is used to get the session data from the server in every post back.
Question 81.
Is Session_End event supported in all session modes?
Answer:
Session_End event occurs only in “InProc mode”. “StateServer” and “SQL Server” do not have Session_End event.
Question 82.
Where do you specify session state mode in ASP.NET?
Answer:
<sessionState mode="SQLServer" stateConnectionString="tcpip=192.168.1.1: 42424" sqlConnectionString="data source=192.168.1.1; Integrated Security=SSPI" cookieless="false" timeout="20" />
Above is sample session state mode specified for SQL Server.
Question 83.
What are the other ways you can maintain state?
Answer:
Other than session variables, you can use the following techniques to store state:
- Hidden fields
- View state
- Hidden frames
- Cookies
- Query strings
Question 84.
What are benefits and limitation of using hidden fields?
Answer:
Following are the benefits of using hidden fields:
- They are simple to implement.
- As data is cached on the client-side, they work with Web Farms.
- All browsers support hidden fields.
- No server resources are required.
Following are the limitations of hidden field:
- They can be tampered creating a security hole.
- Page performance decreases if you store large data, as the data are stored in pages themselves.
- Hidden fields do not support rich structures as HTML hidden fields are only single-valued. Then you have to work around with delimiters, etc., to handle complex structures.
Below is how you will actually implement hidden field in a project.
<input id=”HiddenValue”type=”hidden”value=”lnitial Value”rur>at=”server”NAME-”HiddenValue”>
Question 85.
What is ViewState?
Answer:
ViewState is a built-in structure for automatically retaining values between multiple requests for the same page. ViewState is internally maintained as a hidden field on the page but is hashed, providing greater security than developer-implemented hidden fields do.
Question 86.
How do we ensure ViewState has not tampered?
Answer:
ViewState is a simple HTML hidden field. So it’s possible very much possible that some one can tamper this hidden field easily. To ensure that ViewState is not tampered we can set the “EnableviewStateMac” attribute to true. This attribute is found in the “Page” directive in ASPX page. Below is a simple code snippet which shows how to use the same.
<%@ Page EnableViewStateMac=”true” %>
Question 87.
Does the performance for ViewState vary according to User controls?
Answer:
Performance of ViewState varies depending on the type of server control to which it is applied. Label, TextBox, CheckBox, RadioButton, and HyperLink are server controls that perform well with ViewState. DropDownList, ListBox, DataGrid, and DataList suffer from poor performance because of their size and the large amounts of data making roundtrips to the server.
Question 88.
What are benefits and limitation of using ViewState for state management?
Answer:
Following are the benefits of using ViewState:
- No server resources are required because state is in a structure in the page code.
- Simplicity.
- States are retained automatically.
- The values in ViewState are hashed, compressed, and encoded, thus representing a higher state of security than hidden fields.
- ViewState is good for caching data in Web frame configurations because the data is cached on
the client.
Following are limitation of using ViewState:
- Page loading and posting performance decreases when large values are stored because ViewState is stored in the page.
- Although view state stores data in a hashed format, it can still be tampered because it is stored in a hidden field on the page. The information in xhe hidden field can also be seen if the page output source is viewed directly, creating a potential security risk.
Below is sample of storing values in ViewState. this. ViewState[“EnterTime”] = DateTime.Now. ToStringQ;
Question 89.
How can you use hidden frames to cache client data?
Answer:
This technique is implemented by creating a Hidden frame in page which will contain your data to be cached.
<FRAMESET cols="100%, *, *"> <FRAMESET rows="100%"> <FRAME src="data_of_f ramel .html"x/FRAMESET> <FRAME src="data_of_hidden_frame.html"> <FRAME src="data_of_hidden_frame . html " frameborder="0 " noresize scrolling="yes"> </FRAMESET>
Above is a sample of hidden frames where the first frame “data_of_frame1.html” is visible and the remaining frames are hidden by giving whole cols section to first frame. 100% is allocated to first frame and remaining frames thus remain hidden.
Question 90.
What are benefits and limitations of using Hidden frames?
Answer:
Following are the benefits of using hidden frames:
- You can cache more than one data field.
- The ability to cache and access data items stored in different hidden forms.
- The ability to access JScript® variable values stored in different frames if they come from the same site.
The limitations of using hidden frames are:
- Hidden frames are not supported on all browsers.
- Hidden frames data can be tampered thus creating security hole.
Question 91.
What are benefits and limitations of using Cookies?
Answer:
Following are benefits of using cookies for state management:
- No server resources are required as they are stored in client.
- They are lightweight and simple to use.
Following are limitation of using cookies:
- Most browsers place a 4096 byte limit on the size of a cookie, although support for 8192 byte cookies is becoming more common in the new browser and client-device versions available today.
- Some users disable their browser or client device’s ability to receive cookies, thereby limiting the use of cookies.
- Cookies can be tampered and thus creating a security hole.
- Cookies can expire thus leading to inconsistency.
Below is sample code of implementing cookies
Request. Cookies.Add(New HTTPCookie(“name”, “user1 ”))
Question 92.
What is query string and what are benefits and limitations of using query strings?
Answer:
A query string is information sent to the server appended to the end of a page URL (Uniform Resource Locator).
Following are the benefits of using query string for state management:
- No server resources are required. The query string containing in the HTTP requests for a specific URL.
- All browsers support query strings.
Following are limitations of query string:
- Query string data is directly visible to user thus leading to security problems.-
- Most browsers and client devices impose a 255 character limit on URL length.
Below is a sample “Login” query string passed in URL
HTTP: //www.querystring.com/login.asp?login=testing.
This query string data can then be requested later by using Request. Querystring (“login”).
Question 93.
What are absolute expiration and sliding expiration?
Answer:
Absolute expiration allows you to specify the duration of the cache, starting from the time the cache is activated. The following example shows that the cache has a cache dependency specified, as well as an expiration time of one minute:
Cache. lnsert("announcement”, announcement, depends, DateTime. Now.AddMinutes( 1), Nothing)
Sliding expiration specifies that the cache will expire if a request is not made within a specified duration. The sliding expiration policy is useful whenever you have a large number of items that need to be cached because this policy enables you to keep only the most frequently accessed items in memory. For example, the following code specifies that the cache will have a sliding duration of one minute. If a request is made 59 seconds after the cache is accessed, the validity of the cache would be reset to another minute:
Cache.Insert("announcement", announcement, depends, _ DateTime.MaxValue, _ TimeSpan.FromMinutes(1))
Question 94.
What is cross page posting?
Answer:
Note: This is a feature in ASP.NET2.0
By default, button controls in ASP.NET pages post back to the same page that contains the button, where you can write an event handler for the post. In most cases this is the desired behavior, but occasionally you will also want to be able to post to another page in your application. The Server. Transfer method can be used to move between pages, however the URL does not change. Instead, the cross page posting feature in ASP.NET 2.0 allows you to fire a normal post back to a different page in the application. In the target page, you can then access the values of server controls in the source page that initiated the post back.
To use cross page posting, you can set the PostBackurl property of a Button, LinkButton or ImageButton control, which specifies the target page. In the target page, you can then access the Previous Page property to retrieve values from the source page. By default, the Previous Page property is of type Page, so you must access controls using the FindControi method. You can also enable strongly-typed access to the source page by setting the @PreviousPageType directive in the target page to the virtual path or Type name of the source page. Here is a systematic guide for implementing the cross-page post back using controls that implement the iButtonControi interface.
- Create a Web Form and insert a Button control on it using the VS .NET designer.
- Set the button’s PostBackUrl property to the Web Form you want to post back. For instance in this case it is “nextpage.aspx”
<asp: Button ID="Button 1" runat=”server” PostBackUri=”~/nextpage.aspx” Text-”Post to nextpage”/>
When the PostBackurl property of the IButtonControi is set, the ASP.NET framework binds the corresponding HTML element to new JavaScript function named WebForm_DoPostBackwithOptions. The corresponding HTML rendered by theASP.NET 2.0 will look like this.
<input type="submit" name="Buttonl" value="Post to Page 2" onclick="javascript: WebForm_DoPostBackWithOptions(new WebForm_PostBackOptions("Buttonl", ", false", "Page2.aspx", false, false))" id="Buttonl" />
Question 95.
How do we access ViewState value of the current page in the next page?
Answer:
ViewState is page specific; it contains information about controls embedded on the particular page.
ASP.NET 2.0 resolves this by embedding a hidden input field name, POSTBACK. This field is
embedded only when there is an IButtonControi on the page and its PostBackurl property is set to a non-null value. This field contains the ViewState information of the poster page. To access the ViewState of the poster page, you can use the new PreviousPage property of the page:
Page poster = this. PreviousPage;
Label posterLabel = poster. findControl(“myLabel”); string Ibl = posterLabel. Text;
This cross page post back feature also solves the problem of posting a Form to multiple pages, because each control, in theory, can point to different post back URL.
Question 96.
Can we post and access ViewState in another ASP.NET page?
Answer:
You cannot access ViewState in other pages directly. You can use the previous page property of post back if you want to access.
Question 97.
What is SQL Cache Dependency in ASP.NET 2.0?
Answer:
SQL Cache dependency helps to cache tables in ASP.NET application in memory. So rather than making SQL server trips we can fetch the data from the cached object from ASP.NET.
Question 98.
How do we enable SQL Cache Dependency in ASP.NET 2.0?
Answer:
Below are the broader steps to enable a SQL Cache Dependency:
- Enable notifications for the database.
- Enable notifications for individual tables.
- Enable ASP.NET polling using the “Web.config” file.
- Finally, use the Cache dependency object in your ASP.NET code.
Enable notifications for the database
Before you can use SQL Server cache invalidation, you need to enable notifications for the database. This task is performed with the aspnet_regsql.exe command-line utility, which is located in the c: \[WinDir]\Microsoft.NET\Framework\[Version] directory.
aspnet_regsql -ed -E -d Northwind
-ed: command-line switch -E: Use trusted connection -S: Specify server name it other than the current computer you are working on -d: Database Name
So now, let us try to understand what happens in the database because of “aspnet_regsql.exe”. After we execute the “aspnet_regsql -ed -E -d Northwind” command you will see one new table and four new stored procedures created.
Essentially, when a change takes place, a record is written in this table as shown in Figure 5.23. The SQL Server polling queries this table for changes.
Just to make brief run of what the stored procedures do as shown in Figure 5.24.
“AspNet_SqlCacheRegisterTableStoredProcedure”: This stored procedure sets a table to support notifications. This process works by adding a notification trigger to the table, which will fire when any row is inserted, deleted, or updated.
“AspNet_SqlCacheUnRegisterTableStoredProcedure”: This stored procedure takes a registered table and removes the notification trigger so that notifications won’t be generated.
“AspNet_SqlCachellpdateChangeldStoredProcedure”: The notification trigger calls this stored procedure to update the AspNet_SqlCacheTablesForChangeNotification table, thereby indicating that the table has been changed.
AspNet_SqlCacheQueryRegisteredTablesStoredProcedure: This extracts just the table names from the AspNet_SqlCacheTablesForChangeNotification table. It is used to get a quick look at all the registered tables.
AspNet_SqlCachePollingStoredProcedure: This will get the list of changes from the AspNet_SqlCacheTablesForChangeNotification table. It is used to perform polling.
Enabling notification for individual tables
Once the necessary stored procedure and tables are created then we have to notify saying which table needs to be enabled for notifications.
That can be achieved by two ways:
- aspnet_regsql -et -E -d Northyvind -t Products
- Exec spNet_SqlCacheRegisterTableStoredProcedure ‘TableName’
Registering tables for notification internally creates trigger for the tables. For instance, for a “Products” table the following trigger is created. So any modifications done to the “Products” table will update the “AspNet_SqlCacheNotification’ table.
CREATE TRIGGER
dbo.[Products_AspNet_SqlCacheNotification_Trigger] ON [Products] FOR INSERT, UPDATE, DELETE AS BEGIN SET NOCOUNT ON EXEC dbo.AspNet_SqlCacheUpdateChangeIdStoredProcedure N'Products' END
“AspNet_SqlCacheTablesForChangeNotification” contains a single record for every table you’re monitoring. When you make a change in the table (such as inserting, deleting or updating a record), the changeld column is incremented by 1 as shown in Figure 5.25. ASP.NET queries this table repeatedly keeps track of the most recent changed values for every table. When this value changes in a subsequent read, ASP.NET knows that the table has been changed.
For programmatic data caching, we need to create a new SqiCacheDependency and supply that to the Cache . Insert () method. In the SqiCacheDependency constructor, you supply two strings. The first is the name of the database you defined in the element in the section of the Web.config file e.g: Northwind. The second is the name of the linked table e.g: Products.
private static void CacheProductsList(List<ClsProductItem> products) {SqiCacheDependency sqlDependency = new SqiCacheDependency("Northwind", "Products"); HTTPContext.Current.Cache.Insert("ProductsList", products, sqlDependency, DateTime.Now.AddDays(1), Cache.NoSlidingExpiration);} . private static List<ClsProductItem> GetCachedProductList() {return HTTPContext.Current.Cache["ProductsList"] as List<ClsProductItem>;} cisProductitem is business class, and here we are trying to cache a list of cisProductitem instead of DataSet or DataTable. ' The following method is used by an objectDataSource control to retrieve list of products public static List<ClsProductItem> GetProductsList(int catld, string sortBy) { //Try to Get Products List from the Cache List<ClsProductItem> products = GetCachedProductList(); if (products == null) { //Products List not in the cache, so query the Database layer ClsProductsDB db = new ClsProductsDB(_connectionString); DbDataReader reader = null; products = new List<ClsProductItem>(80); if (catld > 0) { //Return Product List from the Data Layer reader = db.GetProductsList(catld); } else { //Return Product List from the Data Layer reader = db.GetProductsList(); } //Create List of Products -List if ClsProductltem- products = BuildProductsList(reader); reader.Close(); //Add entry to products list in the Cache CacheProductsList(products); } products.Sort(new ClsProductltemComparer(sortBy)); if (sortBy.Contains("DESC")) products.Reverse(); return products; }
To perform the same trick with output caching, you simply need to set the sqlDependency property with the database dependency name and the table name, separated by a colon:
<%@ OutputCache Duration=”600“ SqlDependency=’’Northwind: Products” VaryByParam=”none” %>
The same technique works with the SqlDataSource and obj ectDataSource controls:
<asp: SqlDataSource EnableCaching=”True” SqlCacheDependency=”Northwind: Products”... />
Note: ObjectDataSource doesn’t support built in caching for Custom types such as the one in our example. It only supports this feature for DataSets and DataTables.
Just to make a sample check run the SQL Server profiler and see that does the SQL actually hit the database after the first run.
Question 99.
What is post cache substitution?
Answer:
Post cache substitution is used when we want to cache the whole page but be also need some dynamic region inside that cached page. Some examples like QuoteoftheDay, RandomPhotos, and AdRotator, etc., are examples where we can implement post cache substitution.
Post cache substitution can be achieved by two means:
- Call the new Response. writeSubstitution method, passing it a reference to the desired substitution method callback.
- Add a <asp: Substitution> control to the page at the desired location, and set its methodName attribute to the name of the callback method.
You can see we have a static function here “GetDateTostring ()”. We pass the response substitution callback to the “WriteSubstitution” method. So now, whenASP.NET page framework retrieves the cached page, it automatically triggers your callback method to get the dynamic content.
Figure 5.28 shows the substitution control example that appeared in the Toolbox section. It has been using by “WriteSubstitution” as shown in Figure 5.27. Now let’s try to see how we can do by using “<asp: substitution;*” control. You can get the “<asp: substitution;*” control from the Editor Toolbox.
Figure 5.29 shows is a sample code that shows how substitution control works. We have ASPX code at the right hand side and class code at the left hand side. We need to provide the method name in the “methodName” attribute of the substitution control.
Question 100.
Why do we need methods to be static for Post Cache substitution?
Answer:
ASP.NET should be able to call this method even when there is no instance of your page class available. When your page is served from the cache, the page object is not created. Therefore, ASP.NET skips the page life cycle when the page is coming from cache, which means it will not create any control objects or raise any control events. If your dynamic content depends on the values of other controls, you will need to use a different technique, because these control objects will not be available to your callback
Question 101.
How do we encrypt Web.config files in ASP.NET?
Answer:
Encryption can be done in ASP.NET using the “aspnet_regiis.exe” tool. There are two encryption options providedbyASP.NET:
Windows Data Protection API (DPAPI) Provider (DataProtectionConfigurationProvider) – This provider uses the built-in cryptography capabilities of Windows to encrypt and decrypt the configuration sections. By default this provider uses the machine’s key.
RSA Protected Configuration Provider (RSAProtectedConfigurationProvider) – Uses RSA public key encryption to encrypt/decrypt the configuration sections. With this provider you need to create key containers that hold the public and private keys used for encrypting and decrypting the configuration information.
While encrypting the config files we can choose what kind of provider we need for encryption. So let’s understand step-by-step how we can actually encrypt the Web.config file sections.
Step 1: Go to the command prompt of the framework.
Step 2: Run the aspnet_regiis.exe as shown in the Figure 5.30. We have provided the section which we need to encrypt and the provider. If the command is successfully executed, you should get a succeeded message of encryption. You can see we have decrypted the appSettings section. We have also shown how the unencrypted config file looks after running aspnet_regiis.exe.
Step 3: Once the file is encrypted you can use the same in your program in a normal fashion. For instance the below defined AppSettings key “Myvalue” in figure “aspnet_regiis.exe in Action” can be displayed simply by:
Response.Write(WebConfigurationManager.AppSettings(“MyValue”).ToString())
You do not need to do any kind if decryption inside your program again.
Figure 21.4 shows how the plain text is further changed to an encrypted form using aspnet_regiis.exe.
Below is the aspnet_regiis in different forms for your referral.
— Generic form for encrypting the Web.config file for a particular Website… aspnet_regiis.exe -pef section physical_directory -prov provider
— or —
aspnet_regiis.exe -pe section -app virtual_directory -prov provider
— Concrete example of encrypting the Web.config file for a particular Website
aspnet_regiis.exe -pef “connectionStrings” “C: \Inetpub\wwwroot\MySite” – prov “DataProtectionConfigurationProvider”
— or —
aspnet_regiis.exe -pe “connectionStrings” -app “/MyWebSite” -prov “DataProtectionConfigurationProvider”
— Generic form for decrypting the Web.config file for a particular Website… aspnet_regiis.exe -pdf section physical_directory
— or —
aspnet_regiis.exe -pd section -app virtual_directo’ry
— Concrete example of decrypting the Web.config file for a particular Website…
aspnet_regiis.exe -pdf “connectionStrings” “C: \Inetpub\wwwroot\MyWebSite”
— or —
aspnet_regiis.exe -pd “connectionStrings” -app “/MyWebSite”
Question 102.
In .NET 1.X how was the encryption implemented for config files?
Answer:
Encrypting in .NET 1 .X was a bit different and cryptic as compared to ASP.NET 2.0. It is a three step procedure to implement encryption of config files:
Step 1: Use the aspnet_setreg.exe to make a registry entry using the following command, “-k” is the keyname in the registry and ” -c” is the key value.
aspnet_setreg.exe-k: SOFTWARE\Your_Service\SessionState -c: myConnectionString=”DSN=test; uid=test;pwd=test; ”
Step 2: We give the registry path with the key name “myConnectionString” in the value attribute.
<appSettings> <add key="myConnectionString" value="registry: HKLM\SOFTWARE\Your_Service\Sessionstate\ASPNET_SETREG, myConnectionString" />
Step 3: In the code we need to finally decrypt the connection string as shown in Figure 5.31. For that we need to use Ncrypto DLL.
Note: We have provided the same in the CD zipped in “aspnetreg.zip” file. It has the aspnet_setreg.exe and also the Ncrypto DLL. You can use the DLL and practice the same on .NET 1.X
In the code we need to decrypt back the value so that we can get original string in the process. Below code snippet “Decrypting the connectionstring” shows step-by-step how the decryption process happens. In the below code there are four important steps we need to understand.
Step 1: We need to take the path value using the split section. In this Step 2 we have taken out the path using the “:” and “,” separators.
Step 2: Using the “OpenSubKey” function we open a connection to the path which we just obtained from parsing.
Step 3: We get the value in byte array.
Step 4: We decode the byte array back in to a string value using the “Unprotect” function as shown in Figure 5.31.
Note: This is on the marked improvements in ASP.NET 2.0 we do not need to write a decrypt function. In short we just do the encryption using aspnet_regiis.exe and then call then just read the config value.
Question 103.
How do you send an e-mail using ASP.NET?
Answer:
We need to import the ‘System.Web.Mail’ namespace and use SMTP (Simple Mail Transfer Protocol) component for the same. Below is the code snippet for the same.
// create the mail message MailMessage objEmail = new'MailMessage() ; objEmail.To = "shiv_koirala@yahoo.com"; objEmail.From = "shiv_koirala@yahoo.com"; objEmail.Cc = "shiv_koirala@yahoo.com"; objEmail.Subject= "Test Email"; objEmail.Body= "Hi This is test email"; // finally send the email SmtpMail.Send(objEmail);
Question 104.
How did you do deployment and setup in ASP.NET?
Answer:
Below are the steps to prepare deployment and setup in ASP.NET.
Step 1: Click on File -> New Project -> Setup and Deployment -> Web Setup Project.
Step 2: Right click on the. ‘Web Application Folder’ as shown in Figure 5.32 and click ‘Properties Window’. You will be popped with all necessary properties needed to set the IIS Web application property. To understand how the mapping works in the Figure 5.32 we have shown the IIS Website dialog box mapped to the properties of ‘Web Application Folder’ property in the setup.
Figure 5.32: IIS Web Application Properties
Step 3: -> We now need to specify the application whose setup needs to be prepared. So click on File -> Add Existing Project -> Selection the Solution and add it to the IDE (Integrated Development Environment). Now we need to add the output binary files to the setup. So go to the Setup Project -> click Add -> Project Output -> Select Content Files and click OK as shown in Figure 5.33.
Step 4: With the setup we can also need prerequisites like framework, crystal setup or any other supporting setup. This can go as an integrated part in the same setup. To include prerequisite right click on ‘WebSetup’ -> select Properties -> click Prerequisites… as shown in Figure 5.34. Now select which ever prerequisites you want to ship with the application.
Question 105.
What is URL routing?
Answer:
URL routing helps us to define more user friendly names for pages rather than end users getting into physical file names. For example rather than typing HTTP://www.questpond.com/Contactcompanv.aspx. it would make end users life simpler if he/she can type HTTP: //www.questpond.com/Contact.
The benefits of URL routing are as follows:
- Short and sweet URL’s. Users can easily navigate sites without complicated file extensions like .ASPX, .PHP, .CGI, etc.
- URL becomes permanent and they do not change if you change the physical file names.
- You get benefit of search engine optimization as the search engine can now correlate content with file names.
To implement URL routing in ASP.NET is a three-step process (below is the code behind for the same):
- Add “Global.asax” file to yourASP.NET project.
- Import “System.web.Routing” namespace in the “global.asax.es” file (behind code).
- In “Application_Start” event of “Global.asax.es” file use the routes collection to map the URL names with the physical ASPX file names.
Below is a simple sample code of “global.asax.es” file which maps “Home” with “DisplayHome.aspx”.
using System.Web.Routing; // Import namespace namespace WebRouting { public class Global: System.Web.HTTPApplication { protected void Application_Start(object sender, EventArgs e) { RouteTable.Routes.MapPageRoute("Home", "Home", "-/ DisplayHome.aspx"); // Map routes } } }
Question 106.
Explain the difference between ASP.NET Label control and literal control.
Answer:
Label | Literal |
Used to display formatted text on the browser | Used to display HTML code on the browser. |
Display’s inside a span tag. | Displays the text as it is. So if you have HTML it will get displayed as it is and not rendered. |
We can apply formatting and style | There is no formatting and style here whatever is the text gets displayed as it is. |
Can be accessed via JavaScript easily. | Even if you provide ID value you can access via JavaScript. |