In the previous article, we have discussed C++ Program to Convert Decimal Number to Binary Number. In this article, we will see C++ Program to Convert Decimal Number to Octal Number.
C++ Program to Convert Decimal Number to Octal Number
- Write a C++ program to convert decimal to octal number.
- Write a C++ program to convert octal to decimal number.
In below C++ programs we will learn about fundamentals of decimal and octal number system, how to convert decimal numbers to octal numbers and vice-versa. Given a decimal and an octal number we have to convert it to octal and decimal numbers respectively.
Decimal number system is a base 10 number system using digits for 0 to 9 and Octal number system is base 8 number system and uses 0 and 7.
For Example
100 in decimal number is equivalent to 144 in octal number system.
C++ program to convert a decimal to octal number
Algorithm to convert decimal to octal number
- Divide the input decimal number by 8 and store the remainder.
- Store the quotient back to the input number variable.
- Repeat this process till quotient becomes zero.
- Equivalent octal number will be the remainders in above process in reverse order.
For Example:
Suppose input decimal number is 500
Step 1. 500/8 , Remainder = 4, Quotient = 62
Step 2. 62/8 , Remainder = 6, Quotient = 7
Step 3. 7/8 , Remainder = 7, Quotient = 0
Now, the Octal equivalent of 500 is the remainders in reverse order : 764
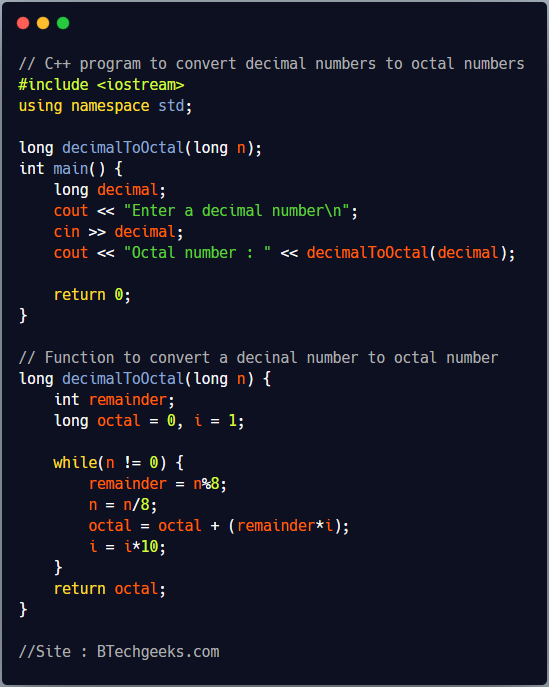
// C++ program to convert decimal numbers to octal numbers
#include <iostream>
using namespace std;
long decimalToOctal(long n);
int main() {
long decimal;
cout << "Enter a decimal number\n";
cin >> decimal;
cout << "Octal number : " << decimalToOctal(decimal);
return 0;
}
// Function to convert a decinal number to octal number
long decimalToOctal(long n) {
int remainder;
long octal = 0, i = 1;
while(n != 0) {
remainder = n%8;
n = n/8;
octal = octal + (remainder*i);
i = i*10;
}
return octal;
}
Output
Enter a decimal number
1234
Octal number : 2322
In above C++ program, we first take an integer as input from user and store it in variable decimal. Then we call decimalToOctal function to convert decimal function to octal number by implementing above mentioned algorithm.
C++ Program to Convert Octal Number to Decimal Number
Algorithm to convert octal to decimal number
- We multiply each octal digit with 8^i and add them, where i is the position of the octal digit(starting from 0) from right side. Least significant digit is at position 0.
Let’s convert 1212(octal number) to decimal number
Decimal number = 1*8^3 + 2*8^2 + 1*8^1 + 2*8^0 = 512 + 128 + 8 + 2 = 650
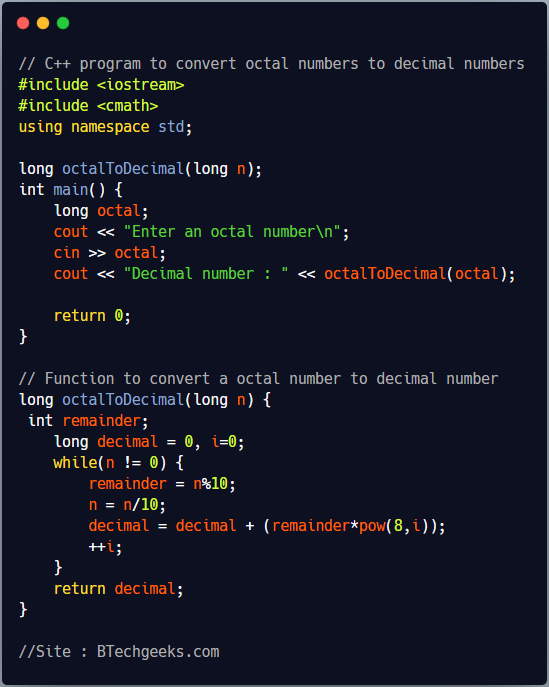
// C++ program to convert octal numbers to decimal numbers
#include <iostream>
#include <cmath>
using namespace std;
long octalToDecimal(long n);
int main() {
long octal;
cout << "Enter an octal number\n";
cin >> octal;
cout << "Decimal number : " << octalToDecimal(octal);
return 0;
}
// Function to convert a octal number to decimal number
long octalToDecimal(long n) {
int remainder;
long decimal = 0, i=0;
while(n != 0) {
remainder = n%10;
n = n/10;
decimal = decimal + (remainder*pow(8,i));
++i;
}
return decimal;
}
Output
Enter an octal number
2322
Decimal number : 1234
In above program, we first take an octal number as input using cin and store it in a long variable octal. Then we call octalToDecimal function by passing octal variable as parameter to convert octal number to decimal number by implementing above mentioned algorithm.
Coding in C++ Programming language helps beginners & expert coders to use the classes for various concepts programs. So, look at the C++ Code Examples of all topics and write a logic with ease.